The Premium C Programming Developer Bundle
109 Enrolled
8 Courses & 84 Hours
$39.99$256.00
You save 84%
What's Included
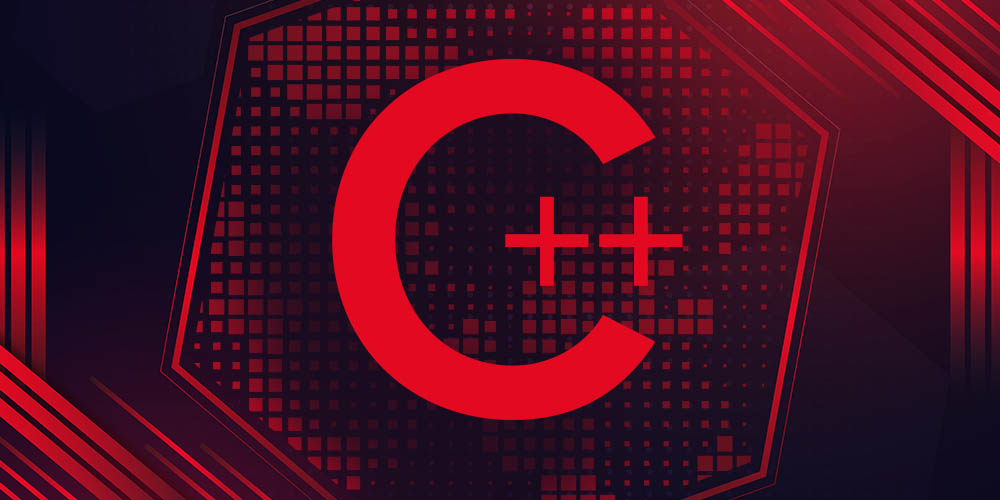
$32.00 Value
Complete Modern C++
Packt Publishing
198 Lessons (19h)
Lifetime
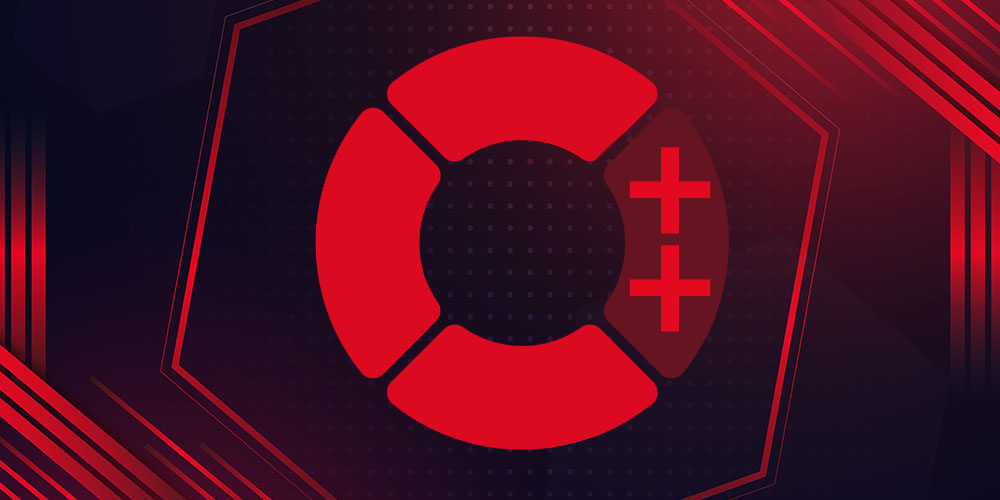
$32.00 Value
Embedded Systems Object-Oriented Programming in C & C++
Packt Publishing
43 Lessons (12h)
Lifetime
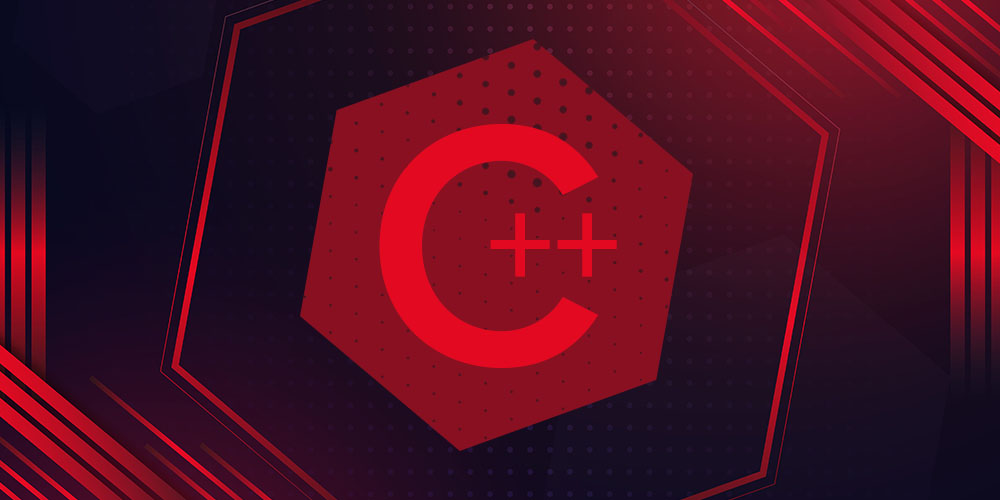
$32.00 Value
Creational Design Patterns in Modern C++
Packt Publishing
43 Lessons (12h)
Lifetime
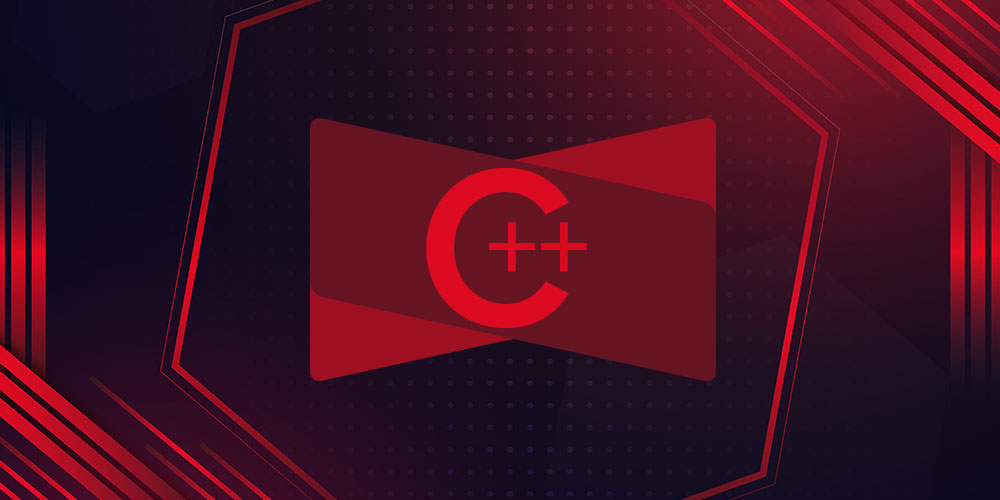
$32.00 Value
The Complete C++ Developer Course
Packt Publishing
107 Lessons (19h)
Lifetime
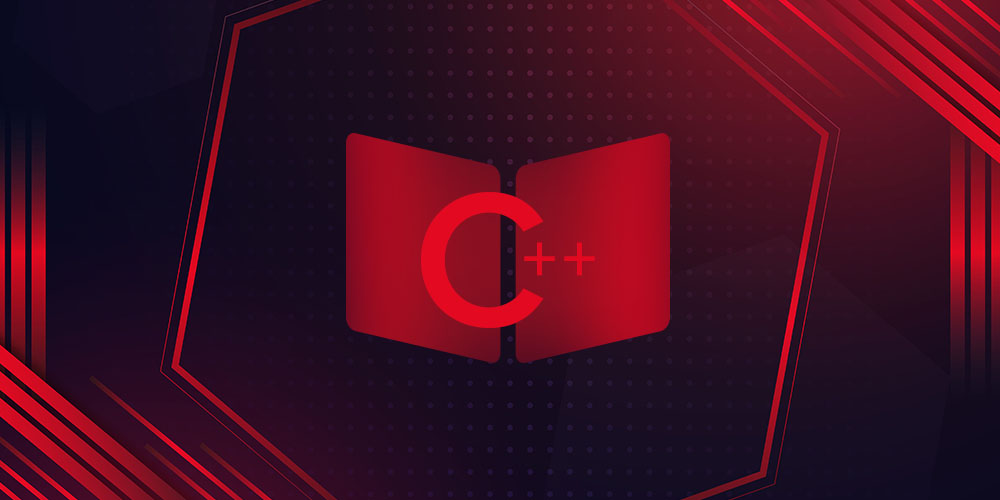
$32.00 Value
Modern C++ Programming Cookbook, Second Edition
Packt Publishing
107 Lessons (19h)
Lifetime
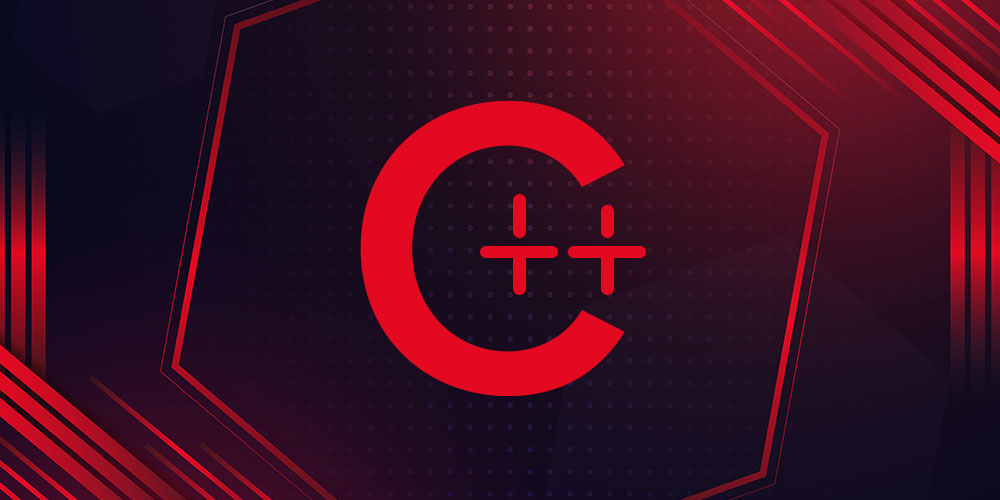
$32.00 Value
C++ High Performance, Second Edition
Packt Publishing
1 Lesson (1h)
Lifetime
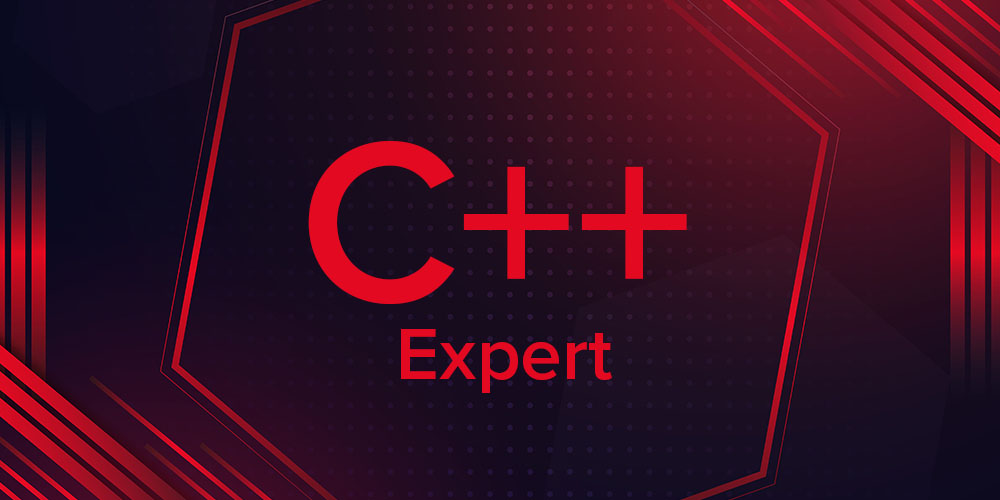
$32.00 Value
Expert C++
Packt Publishing
1 Lesson (1h)
Lifetime
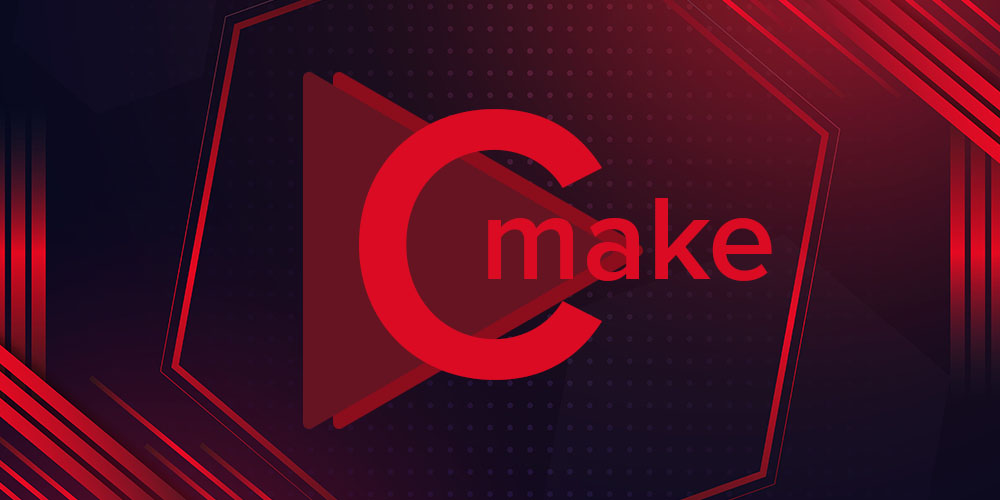
$32.00 Value
Modern CMake for C++
Packt Publishing
1 Lesson (1h)
Lifetime
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
3 Reviews
4.3/ 5
All reviews are from verified purchasers collected after purchase.
TG
Torrey Gragg
Verified Buyer
It's affordability and ease of purchase. It's a great bundle of C++ which makes it easy to learn all i want to know
Jan 28, 2023
IR
Ikbal Rabah
Verified Buyer
Excellent bundle of video courses for any developer who wants to improve his skills in modern C / C++
Dec 21, 2022
CS
CHARLES SIRARD
Verified Buyer
Nice course, nice teacher, well done I will buy other bundle of that kind for sure, i learn a lot, the quality of the sound and the video is great and the website is well made
Dec 11, 2022
Your Cart
Your cart is empty. Continue Shopping!
Processing order...